Please refer to Strings Class 12 Computer Science Important Questions with solutions provided below. These questions and answers have been provided for Class 12 Computer science based on the latest syllabus and examination guidelines issued by CBSE, NCERT, and KVS. Students should learn these problem solutions as it will help them to gain more marks in examinations. We have provided Important Questions for Class 12 Computer Science for all chapters in your book. These Board exam questions have been designed by expert teachers of Standard 12.
Class 12 Computer Science Important Questions Strings
Short Answer Type Questions:
Question: Give the declaration for a string called student, which holds up to 50 characters. Show two ways to initialize the string in the declaration?
Ans: String declaration for student with 50 characters is:
char student[50];
Two methods for declaring strings are:
char student[50+=“Ram\0”;
char student[50]=,‘R’, ‘a’, ‘m’,’\0’-;
Question: What is the limit of char array?
Ans: Char arrays have a special importance in C language. There is not primitive data type to store string data in c. therefore, to store string values in c, we have to use char arrays. Limit (length) of char array defines the length of string. In c clanguage, maximum length of a string in char array can be 65535. Therefore, we can not extend the limit of char array more than 65535.
Question: What is special about the array of char?
Ans: char arrays have special importance in C Language. There is no data type in C to store strings. Therefore, to store string data in, char arrays are being used in C language. To store strings in C, we have to declare char arrays.
For example:
char city[20];
In this example, char array named city has been declared which can store city name with maximum of 20 characters.
Question: List the various functions that can be used to perform the I/O of the string data?
Ans: A string can be read-in or written-out using the input/output functions. Some of the commonly used string input/output functions are explained below:
Reading Strings (String Input Functions):
• scanf() : This function is used to input single word formatted string
• gets() : This function is used to input multiword unformatted string
• getchar() : This function is used to input unformatted single character
Writing Strings (String Output Functions):
• printf() : This function is used to output formatted string
• puts(): This function is used to output unformatted string
• putchar(): This function is used to output unformatted single character
Question: Can we use scanf() to read a string? If no, then give reason. Why it is wrong to use the & symbol when reading in a string with scanf()?
Ans: Yes, we cam use scanf() to read a string. But this function can not be used to inpute multiword strings in C language. There is not data type for storing string data in C. For this, we use char arrays. Name of an array in C represents the address of the first element of array. So, when we use char array in scanf() function, array name itself represents the address of string variable. So, it will be wrong to use the & symbol when reading a string with scanf() function.
Question: What is a difference between “A” and ‘A’?
Ans: “A” is a string while ‘A’ is a character. “A” is string because any string value must be enclosed in double quotes and ‘A’ is char because any char value must be enclosed in single quotes in c language.
Question: Give the difference between putchar() and puts()?
Ans: The difference between putchar() and puts() functions is as follows:
puchar() function: This is the unformatted character output function. This function is used to output a single unformatted character on the monitor screen.
puts() function: This is the unformatted string output function. This function is used to output an unformatted string on the monitor screen.
Question: What is stored in the str, if each of the following values, separately, is read in using a single call to scanf()?
char str[10];
a) cityscape Ans: cityscape
b) New Delhi Ans: New
c) one or more blanks Ans: one
Question: What is string?
Ans: String is a combination of one or more characters. It is always enclosed in double quotes. They are terminated by null character (\0) in c. To store strings in c, we use char array. For example:
char city[20];
It declares a string variable named city. It can store the string of maximum 20 characters.
Consider another example:
char city[20+=”Sunam\0”;
It initializes a string variable named city of length 20 and stores “Sunam” as string value. This value is terminated with null character ‘\0’.
Question: What is the purpose of the null character?
Ans: Null character is also called empty character. It can be represented with the symbol ‘\0’. The length of null character is C is zero. Null character is used to terminate the string in C language. For example:
char city[10]= “Sunam\0”;
It declares a string variable named city of length 10 and stores “Sunam” as string value. This value is terminated with null character ‘\0’.
Question: Explain strcat() function with suitable example.
Ans: Function strcat stands for string concatenation. This function is used to combines the two strings. It appends 2nd string value at the end of first string value. This function is present in the string.h header file. Therefore, to use this function, we have to include the string.h header file in our program. Following code shows how to use this function. Let the two strings are:
char str1*10+=“Sunam”;
char str2*10+=“City”;
strcat(str2, str1);
It adds value of str1 at the end of str2 and make it – CitySunam
Question: Explain strlwr() function with suitable example.
Ans: Function strlwr stands for string lower. This function is used to convert the given string into lower case (i.e. in small letter). This function is present in the string.h header file. Therefore, to use this function, we have to include the string.h header file in our program. Following code shows how to use this function:
strlwr(“PUNJAB”);
It converts the given string into punjab.
Question: Explain strupr() function with suitable example.
Ans: Function strupr stands for string upper. This function is used to convert the given string into Upper case (i.e. in capital letter). This function is present in the string.h header file. Therefore, to use this function, we have to include the string.h header file in our program. Following code shows how to use this function:
strupr(“Punjab”);
It converts the given string into PUNJAB.
Question: Explain atoi () function with suitable example.
Ans: Function atoi stands for ascii to integer. This function converts the string number into integer value. This function is present in the stdlib.h header file. Therefore, to use this function, we have to include the stdlib.h header file in our program. Consider the following example:
atoi(“456”);
It will convert the string number “456” into integer value 456.
Question: Explain strlen() function with suitable example.
Ans: Function strlen() stands for string length. This function is used to count the total number of characters in the given string. This function is present in the string.h header file. Therefore, to use this function, we have to include the string.h header file in our program. Following code shows how to use this function:
strlen(“punjab”);
This function returns 6 as string length because the string “Punjab” contains 6 characters.
Question: Explain strcmp() function with suitable example.
Ans: Function strcmp stands for string compares. The strcmp( ) function is used to compare two strings. It returns one of the three possible values: zero, negative, or positive. It uses ASCII value of characters to compare strings. The function strcmp() is present in the string.h header file. Therefore, to use this function, we have to include the string.h header file in our program. Consider the the following example:
If ASCII values of 1st string is equal to ASCII values of 2nd string, It returns 0
e.g. strcmp(“punjab”,“punjab”) returns 0
If ASCII values of 1st string is less than the ASCII values of 2nd string, It returns negative value
e.g. strcmp(“india”,“punjab”) return –ve value
If ASCII values of 1st string is greater than ASCII values of 2nd string, It returns +ve value
e.g. strcmp(“punjab”,”india”) return +ve value
Question: Explain strrev() function with suitable example.
Ans: Function strrev stands for string reverse. This function is used to reverse the given string value. This function is present in the string.h header file. Therefore, to use this function, we have to include the string.h header file in our program. Following code shows how to use this function:
strrev(“Punjab”);
It reverses the given string to bajnuP.
Question: Explain strcpy() function with suitable example.
Ans: Function strcpy stands for string copy. This function is used to copy a string value into string variable. This function is present in the string.h header file. Therefore, to use this function, we have to include the string.h header file in our program. Following code shows how to use this function.
strcpy(str, “Punjab”);
It copy/store the string value “Punjab” into the string variable str.
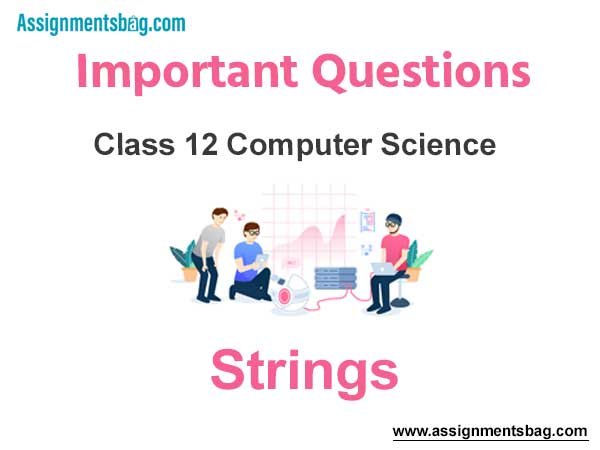